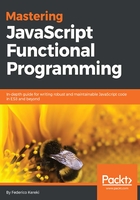
Stubbing
This is a use case similar in some aspects to polyfill: having a function do different work depending on the environment. The idea is to do stubbing, an idea from testing, which means replacing a function with another that does a simpler job, instead of doing the actual work.
A common case is the usage of logging functions. You may want the application to do detailed logging when in development, but not to say a peep when in production. A common solution would be writing something along the lines of:
let myLog = someText => {
if (DEVELOPMENT) {
console.log(someText); // or some other way of logging
} else {
// do nothing
}
}
This works, but as in the example about Ajax detection, it does more work than it
about Ajax detection, it does more work than it needs since it checks every time if the application is in development. We could simplify the code (and get a really, really tiny performance gain!) if we stub out the logging function, so it won't actually log anything:
let myLog;
if (DEVELOPMENT) {
myLog = someText => console.log(someText);
} else {
myLog = someText => {};
}
We can even do better with the ternary operator:
const myLog = DEVELOPMENT
? someText => console.log(someText)
: someText => {};
This is a bit more cryptic, but I prefer it, because it uses a const, which cannot be modified.
Given that JS allows calling functions with more parameters than arguments, and that we aren't doing anything in myLog() when we are not in development, we could have also written () => {} and it would have worked fine. I do prefer, however, keeping the same signature, and that's why I specified the someText argument, even if it wouldn't be used; your call!