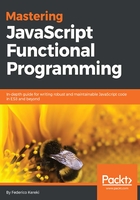
One argument or many?
It's also possible to write functions that return functions, and in Chapter 6, Producing Functions - Higher-Order Functions, we will be seeing more of this. For instance, in the lambda calculus, you don't write functions with several parameters, but only with one, by applying something called currying (why would you do this? Hold that thought; we'll come to that).
Currying gets its name in memory of Haskell Curry, who developed the concept. Note that he is also remembered in the name of an FP language, Haskell; double recognition!
For instance, the function we saw previously that sums three numbers, would be written as follows:
const altSum3 = x => y => z => x + y + z;
Why did I change the function's name? Simply put, because this is not the same function as previously. As is, though it can be used to produce the very same results as our earlier function, it differs in an important way: how do you use it? Say, to sum the numbers 1, 2, and 3? You would have to write:
altSum3(1)(2)(3); // 6
Test yourself before reading on, and mull on this: what would have been returned if you had written altSum3(1,2,3) instead?
Tip: It would not be a number! For the full answer, keep reading.
How does this work? Separating it in many calls can help; this would be the way the previous expression is actually calculated by the JS interpreter:
let fn1 = altSum3(1);
let fn2 = fn1(2);
let fn3 = fn2(3);
Think functionally! The result of calling altSum3(1) is, according to the definition, a function, which in virtue of a closure resolves to be equivalent to:
let fn1 = y => z => 1 + y + z;
Our altSum3() function is meant to receive a single argument, not three! The result of this call, fn1, is also a single argument function. When you do fn1(2), the result is again a function, also with a single parameter, equivalent to:
let fn2 = z => 1 + 2 + z;
And when you calculate fn2(3), a value is finally returned; great! As we said, the function does the same kind of calculations as we had earlier seen, but in an intrinsically different way.
You might think currying is just a peculiar trick: who would want to only use single-argument functions? You'll see the reasons for this, when we consider how to join functions together in Chapter 8, Connecting Functions - Pipelining and Composition, or Chapter 12, Building Better Containers - Functional Data Types, where it won't be feasible to pass more than one parameter from one step to the next.