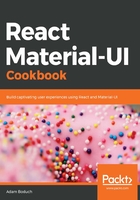
上QQ阅读APP看书,第一时间看更新
How to do it...
Let's say that you have three basic tabs using the following code:
import React, { useState } from 'react';
import { withStyles } from '@material-ui/core/styles';
import Tabs from '@material-ui/core/Tabs';
import Tab from '@material-ui/core/Tab';
const styles = theme => ({
root: {
flexGrow: 1,
backgroundColor: theme.palette.background.paper
}
});
function TabAlignment({ classes }) {
const [value, setValue] = useState(0);
const onChange = (e, value) => {
setValue(value);
};
return (
<div className={classes.root}>
<Tabs value={value} onChange={onChange}>
<Tab label="Item One" />
<Tab label="Item Two" />
<Tab label="Item Three" />
</Tabs>
</div>
);
}
export default withStyles(styles)(TabAlignment);
Here's what you should see when the screen first loads:

By default, tabs are aligned to the left. You can center your tabs by setting the centered property, as follows:
<Tabs value={value} onChange={onChange} centered>
<Tab label="Item One" />
<Tab label="Item Two" />
<Tab label="Item Three" />
</Tabs>
Here's what centered tabs look like:

When your tabs are centered, all of the empty space goes to the left and right of the tabs. The alternative is setting the variant property to fullWidth:
<Tabs value={value} onChange={onChange} variant="fullWidth">
<Tab label="Item One" />
<Tab label="Item Two" />
<Tab label="Item Three" />
</Tabs>
Here's what full width tabs look like:

The tabs are centered, but they're spaced evenly to cover the width of the screen.